Rust's Explicit Error Handling: A Superior Alternative to Try/Catch
Why Rust's Approach to Errors Can Save You from Late-Night Debugging Sessions
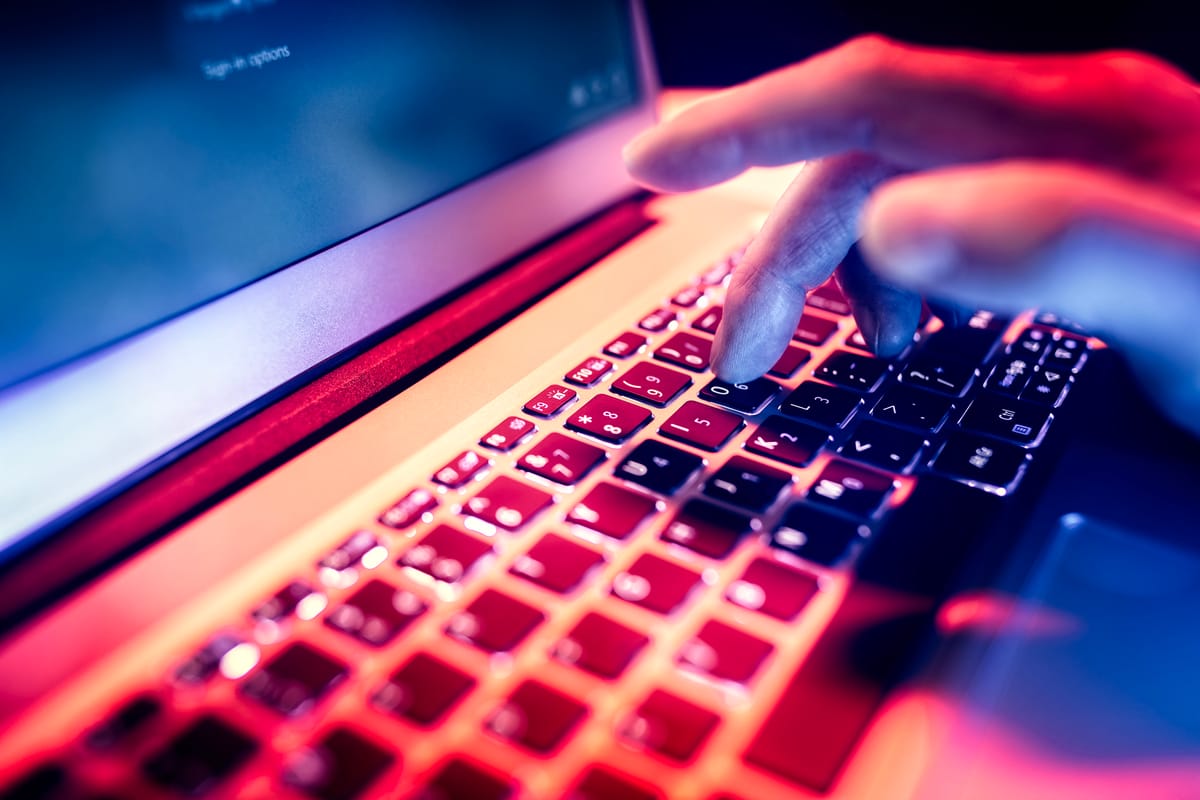
Can you imagine this situation? It’s late on a Friday, and your team is rushing to resolve a production issue. The root cause? A service crashed due to an unhandled exception that bubbled up. This is a scenario that is all too familiar in code bases that rely on implicit error handling. Having worked on large C#, Spring Boot and Node.js systems for years, I have seen many overnight outages and tricky bugs caused by exceptions that were caught too generically, or not at all. Traditional try/catch error handling, while convenient, can obscure the true control flow of a program. The code continues as if nothing went wrong until, suddenly, it doesn’t.
The Hidden Pain of Implicit Error Handling
Implicit error handling through exceptions has well-known pain points.
Exceptions interrupt the normal execution path by jumping to the nearest catch block, which may be located far up the call stack. This non-linear flow makes it difficult to reason about the program's state. Reading a function does not reveal all its exit points if some are via thrown exceptions. It’s easy (and tempting) to catch a broad exception type without distinguishing error causes (e.g. catch (Exception e) in C# or a blanket catch in Java/TS). This results in minimal handling, often just logging, and masks different failure modes under one generic branch.
Even worse, sometimes a catch block simply swallows the error, doing nothing or returning a default value. This causes the program to continue in an undefined state, which can lead to deeper problems. In one Spring Boot postmortem, for example, swallowing an exception meant that the app continued to run in an unexpected state, which could result in further errors and corrupted data.
Since exceptions are not shown in function signatures, they can catch you off guard at runtime. For example, an innocuous method call might throw a NullReferenceException or an I/O error that wasn’t obvious to the caller. Teams often only discover these when an unhandled exception causes something to crash in production. Using exceptions for expected conditions can have a detrimental effect on performance and code.
Exceptions are designed for exceptional cases, so using them for regular control flow is inefficient. Unwinding the stack incurs a cost. In .NET, for instance, excessive exception throwing can reduce throughput. Using exceptions for everyday logic in C# are messy and make your code harder to read. When even Microsoft's own guidelines caution against using exceptions for routine logic, it suggests there is a problem.
These issues have prompted many developers to seek alternatives. From C# to JavaScript, there is a growing realization that implicit error handling (simply throwing and catching errors) is not the most effective approach. In the TypeScript community, for example, there is a great deal of frustration because the try/catch method provides no type safety if any value can be thrown, and the function signature is unaware of what could be thrown. In other words, neither humans nor tools can easily anticipate the errors that a given function might produce. It's a recipe for surprises.
Rust's Approach: Errors as Part of the Control Flow
Rust takes a very different approach to error handling. Rather than using exceptions to handle recoverable errors, Rust encourages us to make error conditions explicit in the type system. A function that might fail will return a Result<T, E> (or an Option<T> if the only 'error' is the absence of a value) instead of throwing an exception. This simple concept is underpinned by the idea that a function’s potential to fail is part of its output and contract.
In languages such as C# and JavaScript, it's easy to overlook potential errors. You call a function and assume it will succeed unless an exception occurs. Rust, by contrast, makes the possibility of failure impossible to ignore. Some languages allow you to ignore potential errors, automatically propagating them as exceptions and causing the program to crash if they are not handled. However, Rust's design is more intentional. A Rust function returns an explicit Result or Option, and this "optionality" or “result-ness” travels with the value. You must either handle the error immediately or propagate it upwards; you cannot simply ignore it. At some point, you must check whether you got the value or an error.
This leads to a kind of enforced discipline. Rust will literally not let you forget to deal with the error case. If you try to use a result without handling the Err, it won’t compile. If you treat an Option<T> as if it were a guaranteed T, Rust will swiftly puncture your unwarranted optimism with a type error. The compiler is your guide, constantly asking you, what about the failure case. In practice, this means fewer unchecked edge cases sneaking through.
It is better to have compile-time checks than runtime surprises. Yes, explicit errors require a little more typing, but that's the trade-off between clarity and convenience. Rust eases that burden with the '?' operator. It is essentially a shorthand for 'if this returns Err, return it now; otherwise unwrap the Ok'.
But this convenience can sometimes work against you. Consider this:
// Imagine fetching an asset might simply not find one.
fn get_asset() -> Option<String> {
None
}
fn get_asset_name() -> Result<String, Box<dyn std::error::Error>> {
let asset = get_asset()?; // ❌ this won’t compile
Ok(asset)
}
You’ll see:
error[E0277]: the `?` operator can only be used on `Result`s, not `Option`s, in a function that returns `Result`
--> src/lib.rs:10:28
|
9 | fn get_asset_name() -> Result<String, Box<dyn std::error::Error>> {
| ------------------------------------- this function returns a `Result`
10 | let asset = get_asset()?;
| ^ use `.ok_or(...)?` to convert the `Option` into a `Result`
Why? Because '?' works on whatever your function returns. In this case, your function returns a Result, so '?' expects a Result. An Option is a different type with no built-in way to become a Result, unless you specify how to map the None case to an error.
The solution is straightforward: if the absence of an asset truly constitutes an error, express it using a Result and explicitly convert the Option.
fn get_asset() -> Result<String, String> {
// ...
Ok("Delivery Van".to_string())
}
fn get_asset_name() -> Result<String, String> {
let asset = get_asset()?; // ✅ compiles and propagates any error
// Do something...
Ok(asset)
}
Both functions now speak the same language: they both return a Result<String, String>. If an asset cannot be found, get_asset() returns an Err("..."), which is automatically bubbled up by get_asset_name().
Another straightforward option is to use a match statement. Since Option is just an enum, you can pattern-match on its variants and handle each case explicitly. In the None arm you return early with an error message; in the Some arm you continue with the value. That makes control flow crystal-clear:
// Simulate fetching an asset; returns None if not found
fn get_asset() -> Option<String> {
None
}
fn get_asset_name() -> Result<String, String> {
// Be explicit: match on the Option, covering both cases
let asset = match get_asset() {
Some(name) => name,
None => return Err("No asset found".into()),
};
// Do something with `asset`…
Ok(asset)
}
Here, the compiler checks that you have handled both 'Some' and 'None'. In the 'None' branch, you immediately return an 'Err', whereas in the 'Some' branch, you bind the value to a name. This method is slightly more verbose than the '?' method, but it clearly explains what is happening at each step, which is useful for learners. Because match is an expression, its final value is assigned to asset.
Use 'match' when you want maximum clarity, especially if you need to perform different actions in each branch. For quick conversions, you can use ? or ok_or, but match is the most explicit tool at your disposal.
Use Option when the absence of an outcome is normal and expected; use Result when the absence of an outcome (or any other failure) is a genuine error. Rust’s compiler won’t silently allow you to mix them up.
It forces you to make your intent clear in the types, rather than hiding it behind a catch.
Fundamentally, Rust’s approach promotes a philosophy of correctness through explicitness. Error handling is not an afterthought or a separate control flow hidden from the type system; it’s woven into the logic of the program. This leads to more robust code. When you see a function signature, you immediately know if it can fail and you need to consider error cases. There’s no need to comb through documentation or implementation to discover “oh, this might throw.” The result is less ambiguity. As an example, consider how obvious it is when a Rust function returns an Option. You must check for None or use a helper, otherwise the compiler will stop you. In contrast, in languages with implicit exceptions, you might call a method without realizing it might throw, or you might forget to surround it with the right try/catch. Rust shifts that knowledge left to compile time.
Conclusion
The transition from implicit error handling languages such as C#, Java and JavaScript to Rust's explicit Result and Option model can initially feel disorientating. It can seem as though you have to type more and think more about errors than you’re used to.
However, this initial extra effort pays off in the form of cleaner, more reliable code. When every possible error is accounted for in the type system, your code paths achieve a certain closure. You either handle an error or pass it on, but you never ignore it. Developers who come from languages with try/catch often have stories about 'exception hell', where debugging is like detective work to find where an error occurred. In Rust, those scenarios are far less common: the compiler supports you from the outset, ensuring you never miss the error handling branch.
And yes I know, I know... .NET 9 introduced a Result<T> type, inspired by Rust, to handle errors without relying heavily on exceptions. Unlike Rust’s compile-time enforcement, the .NET Result<T> uses runtime checks, requiring developers to manually verify the result’s state to avoid errors. While it reduces exception overhead, it lacks Rust’s guarantee of handling errors before the program runs.
Cheers!