Avoiding Over-Engineering: Focus on Real Problems in Software Development
Discover why premature optimization and over-abstraction harm your software projects, and learn a pragmatic approach driven by real-world feedback.
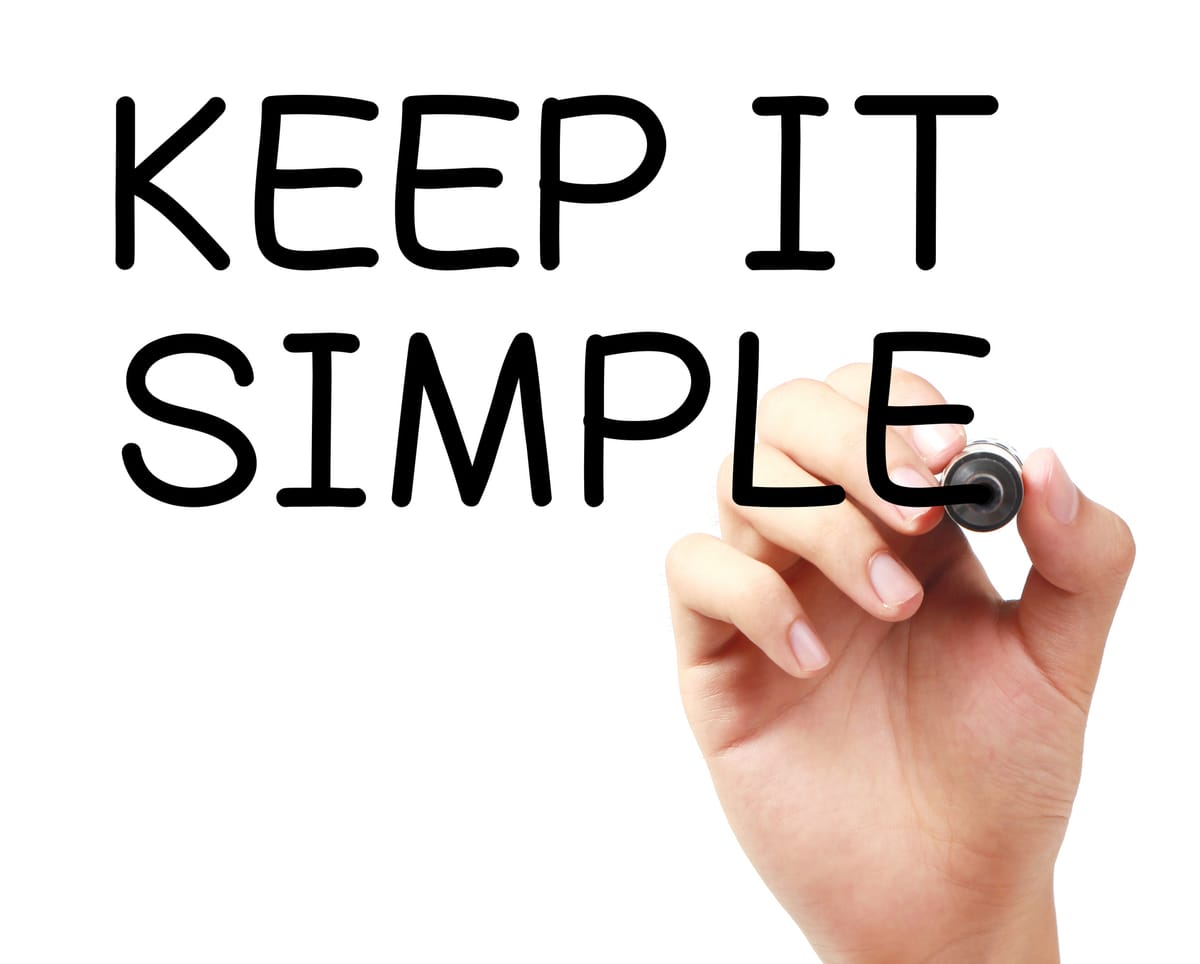
One lesson has hit me repeatedly over the head: we waste a lot of effort trying to solve problems that we don't actually have yet. It’s an easy trap to fall into. We want our code to be lightning fast, perfectly designed and ready for millions of users. All before we've even released anything useful! The intentions are good, but the results can be disastrous. In this article, I’ll break down some of the most common pitfalls of over-engineering that I’ve seen (and committed myself), and I’ll explore a more pragmatic approach that favors real-world feedback over theoretical perfection.
The Cost of Premature Optimization
Computer pioneer Donald Knuth warned that we should forget about small efficiencies 97% of the time, because obsessing over performance too early causes more problems than it solves. I couldn't agree more. Optimizing code before you know where the real bottlenecks are is a classic way to waste time for no good reason.
What does premature optimization look like? It's when you spend days rewriting a function that works well and has already done its job. Believe me, I'm not wagging my finger at you. I've done it myself more than once. It’s pointless to worry about memory usage in a prototype that hasn’t been used by anyone yet. I’ve seen developers (including my younger self) meticulously micro-optimize pieces of code that never even appeared in a CPU profile. The result? Wasted time that could have been spent building features or fixing issues that users actually experience.
The price includes not only time, but also complexity. Hand-optimized code is generally harder to read and maintain. It may rely on clever tricks or special-case logic that future team members (or even you, six months from now) will find hard to understand. And for what? In most cases, these 'optimizations' will have a minimal impact on overall performance. Meanwhile, the real performance hotspots (the ones that really matter!) remain undiscovered because you never tested the application under realistic conditions to identify them.
To be clear, optimization itself isn’t bad. The important thing is to time it right. Use real usage data to profile your application and identify the 3% of code that truly needs to be speeded up. Focus your performance tuning on this code, and keep the other 97% simple. By resisting the urge to make everything 'fast' prematurely, you avoid creating overly complex code that you cannot easily change later. First make it work, then make it fast when necessary.
Over-Abstraction and Premature Generalization
Two related pitfalls in software design are over-abstraction and premature generalization. These often originate from a positive intention, as we aim to write elegant, DRY code and anticipate future requirements. However, if taken too far, they lead to architectures that are far more complicated than necessary.
Over-abstraction is when you add extra layers, classes, or indirection that don't actually solve a problem you have right now. Maybe you create five layers of factories and interfaces to do something very simple, just in case you need to swap out implementations later. Or you build a generic framework for, say, “message processing” when your app only ever needed to handle one type of message. I’ve been there: I once designed an elaborate plugin architecture for a tool that, in the end, had exactly one plugin. All that abstraction was just dead weight.
Premature generalization is similar: it involves writing your code to handle every possible future scenario instead of the one you are currently facing. It’s the “What if we need to do X someday?” mindset. For instance, you might generalize a function to handle an array of inputs when you currently only require one input, or create a polymorphic class hierarchy for three variations of behavior that could be covered by a simple if statement for now.
The result is complex solutions in search of a problem.
Ironically, these efforts to make the code 'future-proof' often make it more difficult to change in the future. In other words, applying an abstraction too soon tends to hinder rather than help maintainability.
How can you avoid this? The key is to plan for the present, not the hypothetical future. Solve the concrete problem you have now in the simplest way possible. If you find yourself writing lots of code 'just in case' or creating layers of indirection without a clear, current need, take a step back. You probably don't need it. This doesn’t mean your code can never evolve; it means you’ll evolve it when you have more information. It's much easier to generalize a simple, working solution later (when the requirements are clearer) than to specialize a bloated, general solution built on guesswork.
Real-World Feedback Trumps Theoretical Architecture
Ultimately, it all comes down to a simple idea: you learn far more from real-world feedback than you do from perfecting an architecture in isolation. In theory, a design can appear perfect. It handles every case elegantly, can be scaled infinitely, and adheres to all best practices. On the whiteboard or in your head, it's perfect. However, as every experienced engineer knows, no application survives first contact with real users.
Users do unexpected things. Requirements change once people start using the software. Bottlenecks appear in unexpected places. By releasing a working product or feature sooner, you can observe these issues and make adjustments. If you delay the release by months or even years while chasing the theoretically perfect design, you’re essentially flying blind the whole time. You’ll make investments that might turn out to be misguided.
In my experience, projects that succeeded embraced an iterative, feedback-driven approach. We would build a simple version, present it to users or testers, and learn from its performance. This feedback would tell us where to strengthen the design or where our assumptions were incorrect. Conversely, projects in which we attempted to plan for every eventuality from the outset, involving extensive design phases and complex architectural diagrams for hypothetical edge cases, tended to fail. We either realized too late that the architecture did not meet the needs of the users, or we had to discard half of it because our assumptions were disproven by reality.
Don't worship an architectural diagram just because it looks good. Favor an architecture that emerges from actual requirements and usage patterns.
Start with something simple that works.
Monitor it, profile it and gather user feedback. Then, where you see real pain points or growth needs, refactor and extend the architecture. This way, every bit of complexity you add is based on evidence that it is needed.
Scaling Fantasies vs. Actual User Growth
A particularly common imaginary problem is the 'we need to scale up to millions of users' fantasy. Engineers love to daydream about systems on a cosmic scale, handling Netflix-level traffic or designing the backend for the next global social network is fun to think about. The result is that teams engineer their software preemptively to handle insane loads and complexity that they will most likely never see, or at least not for a long time.
What dangers are there? Firstly, over-engineering for scale can be fatal before you even acquire a single user. If you’re a startup or developing a new product, complexity is your enemy. In the past, I’ve seen startups waste months building a microservices architecture complete with multiple databases, queues and caches, just to handle traffic levels that only existed in their imagination. Meanwhile, they didn’t focus enough on creating a product that people actually wanted to use. This is a tragically common scenario: by the time they realize this, the money or motivation has run out and the fancy architecture has no real users to justify it.
Even in established companies, I’ve seen teams introduce unnecessary complexity 'for future scalability' that never materialized. For example, we might split a service into dozens of tiny services or adopt an eventually consistent distributed datastore, all under the assumption that huge load is coming. In reality, the load might peak at just 5% of the predicted amount, and a simpler monolithic system would have handled that easily (with far fewer operational headaches).
There is data to support this on the business side as well: studies have found that premature scaling is a leading cause of start-up failure. One large-scale survey concluded that 70% of failed startups had scaled up too early in terms of staffing, spending or technology before achieving product-market fit. Not a single startup that scaled prematurely in that study ever reached 100,000 users. The lesson is that scaling too soon doesn’t just waste effort. It can actively prevent you from ever needing to scale up at all.
So what should you do instead? Scale progressively, in step with real growth. Start with a simple architecture that can handle your current needs plus a bit of headroom. Focus on acquiring users and delivering value. If you’re lucky enough to see rapid user growth, that’s a good problem, and you’ll have actual usage patterns and metrics to guide the scaling efforts. At that point, you can start identifying bottlenecks and refactoring the architecture to handle more load. By then, you’ll also hopefully have more engineers and resources to do it properly.
Remember that many successful tech companies started out with very simple architectures. AirBnb, for example, started out as a monolithic Ruby on Rails application. Facebook began life as a PHP site backed by a single database. They certainly experienced growing pains, but they solved them as they arose, with a clear idea of what needed fixing. It's much better to handle scaling issues as they arise than to try to predict them all in advance.
Evolving Architecture as You Go
By now, it should be clear that I’m a big fan of progressive, evolutionary architecture, which involves designing your system step by step and being guided by real needs:
1) This doesn’t mean “no architecture” or simply piecing things together ad hoc.
2) It means having a vision, but being flexible and willing to adapt as you learn more.
Think of your architecture as a living thing that grows alongside your application. Early on, you keep it lean and flexible. You avoid locking yourself into heavy patterns or one-size-fits-all edicts. As the system matures and you become more confident about certain requirements, e.g. 'we really do need multi-region redundancy' or 'this module is clearly a performance hotspot', you invest in those areas.
One approach that embraces this idea is pragmatic architecture. The idea is to build the simplest thing that could possibly work, prove it out, and then enhance it. This is similar to the concept of evolutionary architecture, whereby the design supports incremental, guided change over time. You can start with a straightforward design and refine it through successive iterations. Each iteration is informed by real-world issues: perhaps you notice that database lock contention is increasing, so you introduce read replicas. Alternatively, users may request a new capability, prompting you to refactor part of the codebase to be more modular.
The benefit is that you avoid taking big risks with unproven requirements. You always solve the most pressing problems, so your engineering effort directly creates user or business value. Meanwhile, you keep technical debt in check because you’re not developing unnecessary features.
Yes, there’s a risk that if you hit it big and your simple design needs a major overhaul, you might have to do some heavy lifting further down the line.
But that would be a first-world problem!
It would mean that you had succeeded enough to warrant a re-architecture. By that time, you'll have a much clearer idea of what the new architecture needs to achieve, and you'll probably have the necessary funding and time to implement it properly. In contrast, if you do too much up front, you might never reach that level of success.
Cheers!